What Level Of Behaviors Deals With Creating Multiple Instances Of A Service To Process Requests?

What is a Design Pattern?
Design patterns are design level solutions for recurring problems that we software engineers come across often. It'south not code - I echo, ❌ Code . Information technology is like a clarification on how to tackle these problems and design a solution.
Using these patterns is considered skillful practise, as the pattern of the solution is quite tried and tested, resulting in higher readability of the last code. Blueprint patterns are quite oftentimes created for and used past OOP Languages, like Java, in which nearly of the examples from here on will be written.
Types of blueprint patterns
There are near 26 Patterns currently discovered (I inappreciably call up I will do them all…).
These 26 can be classified into 3 types:
i. Creational: These patterns are designed for class instantiation. They can be either course-creation patterns or object-creational patterns.
2. Structural: These patterns are designed with regard to a class's structure and composition. The chief goal of virtually of these patterns is to increase the functionality of the class(es) involved, without changing much of its composition.
three. Behavioral: These patterns are designed depending on how one class communicates with others.
In this mail, we will get through one basic design design for each classified type.
Type 1: Creational - The Singleton Design Pattern
The Singleton Blueprint Pattern is a Creational pattern, whose objective is to create only one instance of a class and to provide only one global access point to that object. One commonly used example of such a class in Java is Calendar, where you lot cannot make an instance of that class. It also uses its ain getInstance()
method to become the object to be used.
A class using the singleton blueprint blueprint will include,

- A private static variable, property the but instance of the class.
- A private constructor, and so it cannot be instantiated anywhere else.
- A public static method, to return the unmarried instance of the grade.
At that place are many different implementations of singleton design. Today, I'll be going through the implementations of;
i. Eager Instantiation
2. Lazy Instantiation
3. Thread-safe Instantiation
Eager Beaver
public course EagerSingleton { // create an instance of the form. private static EagerSingleton instance = new EagerSingleton(); // private constructor, then information technology cannot be instantiated outside this class. individual EagerSingleton() { } // get the only instance of the object created. public static EagerSingleton getInstance() { return case; } }
This type of instantiation happens during class loading, as the instantiation of the variable case happens outside any method. This poses a hefty drawback if this class is not being used at all by the client application. The contingency plan, if this class is not existence used, is the Lazy Instantiation.
Lazy Days
At that place isn't much deviation from the above implementation. The main differences are that the static variable is initially declared null, and is only instantiated within the getInstance()
method if - and simply if - the instance variable remains null at the time of the bank check.
public course LazySingleton { // initialize the instance as null. private static LazySingleton case = naught; // private constructor, so information technology cannot be instantiated outside this class. private LazySingleton() { } // check if the instance is null, and if and so, create the object. public static LazySingleton getInstance() { if (instance == zilch) { instance = new LazySingleton(); } render instance; } }
This fixes one problem, only another one still exists. What if two different clients access the Singleton form at the same time, correct to the millisecond? Well, they will bank check if the instance is null at the same time, and will notice it true, and and so will create two instances of the class for each request by the ii clients. To set up this, Thread Safe instantiation is to be implemented.
(Thread) Rubber is Primal
In Java, the keyword synchronized is used on methods or objects to implement thread rubber, so that only one thread will access a particular resource at 1 time. The form instantiation is put within a synchronized block so that the method can only be accessed by one client at a given fourth dimension.
public class ThreadSafeSingleton { // initialize the instance as null. private static ThreadSafeSingleton instance = zero; // individual constructor, then it cannot exist instantiated outside this class. private ThreadSafeSingleton() { } // check if the instance is goose egg, within a synchronized block. If so, create the object public static ThreadSafeSingleton getInstance() { synchronized (ThreadSafeSingleton.course) { if (instance == null) { instance = new ThreadSafeSingleton(); } } return example; } }
The overhead for the synchronized method is loftier, and reduces the performance of the whole operation.
For example, if the instance variable has already been instantiated, then each time whatsoever client accesses the getInstance()
method, the synchronized
method is run and the performance drops. This just happens in order to check if the example
variables' value is null. If it finds that information technology is, it leaves the method.
To reduce this overhead, double locking is used. The bank check is used before the synchronized
method besides, and if the value is null lone, does the synchronized
method run.
// double locking is used to reduce the overhead of the synchronized method public static ThreadSafeSingleton getInstanceDoubleLocking() { if (instance == null) { synchronized (ThreadSafeSingleton.class) { if (instance == zippo) { instance = new ThreadSafeSingleton(); } } } return case; }
At present onto the next nomenclature.
Type 2: Structural - The Decorator Pattern Pattern
I'g gonna give you lot a small scenario to give a meliorate context to why and where you should use the Decorator Design.
Say you own a java store, and similar whatsoever newbie, you starting time out with just two types of plain coffee, the house alloy and nighttime roast. In your billing system, at that place was one class for the different coffee blends, which inherits the beverage abstract class. People actually beginning to come past and accept your wonderful (albeit bitter?) java. Then there are the coffee newbs that, God forbid, want sugar or milk. Such a travesty for coffee!! ??
At present yous demand to have those ii add-ons likewise, both to the menu and unfortunately on the billing system. Originally, your IT person will make a subclass for both coffees, one including sugar, the other milk. And so, since customers are always right, ane says these dreaded words:
"Can I get a milk coffee, with saccharide, please?"
???
There goes your billing system laughing in your face once again. Well, back to the cartoon board….
The Information technology person then adds milk coffee with carbohydrate equally another subclass to each parent coffee class. The residuum of the month is smooth sailing, people lining up to have your java, you actually making money. ??
Simply wait, at that place'due south more!
The earth is against you over again. A competitor opens up across the street, with not just 4 types of java, just more than 10 add together-ons likewise! ?
You buy all those and more than, to sell better coffee yourself, and only then retrieve that you forgot to update that dratted billing system. You quite possibly cannot make the infinite number of subclasses for any and all combinations of all the add-ons, with the new coffee blends besides. Non to mention, the size of the terminal organization.??
Time to actually invest in a proper billing arrangement. Y'all find new It personnel, who actually knows what they are doing and they say;
"Why, this will be so much easier and smaller if it used the decorator pattern."
What on globe is that?
The decorator blueprint pattern falls into the structural category, that deals with the actual structure of a class, whether is by inheritance, composition or both. The goal of this design is to modify an objects' functionality at runtime. This is one of the many other design patterns that utilize abstruse classes and interfaces with composition to get its desired consequence.
Permit's requite Math a chance (shudder?) to bring this all into perspective;
Take 4 coffee blends and x add-ons. If we stuck to the generation of subclasses for each unlike combination of all the add-ons for ane type of coffee. That'southward;
(ten–1)² = 9² = 81 subclasses
We subtract one from the 10, every bit you lot cannot combine ane add together-on with another of the same type, sugar with sugar sounds stupid. And that's for just 1 java alloy. Multiply that 81 by 4 and you get a whopping 324 unlike subclasses! Talk nigh all that coding…
Merely with the decorator blueprint will crave just 16 classes in this scenario. Wanna bet?
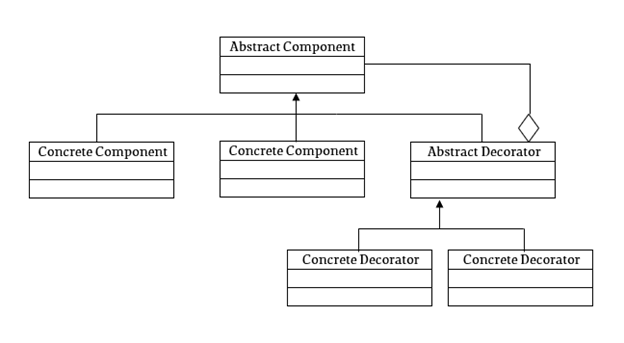
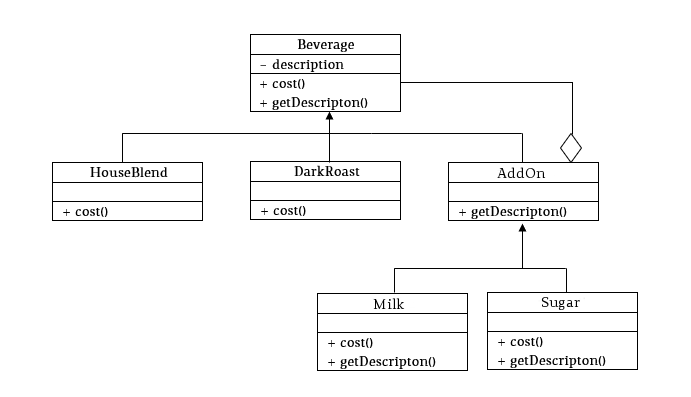
If we map out our scenario according to the class diagram higher up, we get four classes for the 4 coffee blends, 10 for each addition and 1 for the abstract component and 1 more for the abstract decorator. Come across! 16! Now paw over that $100.?? (jk, just it will non be refused if given… just maxim)
As you can see from above, just every bit the concrete coffee blends are subclasses of the potable abstract class, the AddOn abstract class also inherits its methods from it. The add-ons, that are its subclasses, in plow inherit whatsoever new methods to add functionality to the base of operations object when needed.
Permit'southward go to coding, to see this pattern in employ.
First to make the Abstract beverage grade, that all the different coffee blends will inherit from:
public abstract form Beverage { private Cord clarification; public Drink(String clarification) { super(); this.description = description; } public String getDescription() { render clarification; } public abstract double price(); }
And then to add both the concrete coffee blend classes.
public grade HouseBlend extends Potable { public HouseBlend() { super("House blend"); } @Override public double cost() { return 250; } } public class DarkRoast extends Beverage { public DarkRoast() { super("Dark roast"); } @Override public double toll() { render 300; } }
The AddOn abstract form as well inherits from the Beverage abstract class (more on this beneath).
public abstract grade AddOn extends Beverage { protected Beverage beverage; public AddOn(String description, Beverage bev) { super(description); this.beverage = bev; } public abstract String getDescription(); }
And now the concrete implementations of this abstract class:
public class Saccharide extends AddOn { public Sugar(Beverage bev) { super("Carbohydrate", bev); } @Override public String getDescription() { render beverage.getDescription() + " with Mocha"; } @Override public double cost() { return drinkable.price() + fifty; } } public class Milk extends AddOn { public Milk(Beverage bev) { super("Milk", bev); } @Override public String getDescription() { return potable.getDescription() + " with Milk"; } @Override public double cost() { return beverage.price() + 100; } }
As y'all tin see higher up, we tin can laissez passer any subclass of Beverage to whatsoever subclass of AddOn, and get the added toll equally well as the updated description. And, since the AddOn class is essentially of type Drinkable, we tin pass an AddOn into another AddOn. This way, we can add whatever number of add together-ons to a specific coffee blend.
Now to write some code to test this out.
public class CoffeeShop { public static void main(String[] args) { HouseBlend houseblend = new HouseBlend(); System.out.println(houseblend.getDescription() + ": " + houseblend.cost()); Milk milkAddOn = new Milk(houseblend); Organisation.out.println(milkAddOn.getDescription() + ": " + milkAddOn.cost()); Sugar sugarAddOn = new Sugar(milkAddOn); Arrangement.out.println(sugarAddOn.getDescription() + ": " + sugarAddOn.cost()); } }
The concluding result is:
It works! We were able to add more one add-on to a coffee alloy and successfully update its terminal cost and description, without the need to make infinite subclasses for each improver combination for all java blends.
Finally, to the concluding category.
Blazon three: Behavioral - The Command Design Pattern
A behavioral design pattern focuses on how classes and objects communicate with each other. The main focus of the command pattern is to inculcate a higher degree of loose coupling between involved parties (read: classes).
Uhhhh… What's that?
Coupling is the way that ii (or more) classes that interact with each other, well, interact. The platonic scenario when these classes interact is that they exercise non depend heavily on each other. That'due south loose coupling. Then, a better definition for loose coupling would be, classes that are interconnected, making the to the lowest degree utilise of each other.
The need for this pattern arose when requests needed to be sent without consciously knowing what yous are asking for or who the receiver is.
In this pattern, the invoking form is decoupled from the class that actually performs an action. The invoker grade only has the callable method execute, which runs the necessary control, when the client requests it.
Permit's take a basic real-world example, ordering a repast at a fancy restaurant. As the menstruum goes, yous give your order (command) to the waiter (invoker), who then hands it over to the chef(receiver), so you can go food. Might sound unproblematic… merely a chip meh to lawmaking.
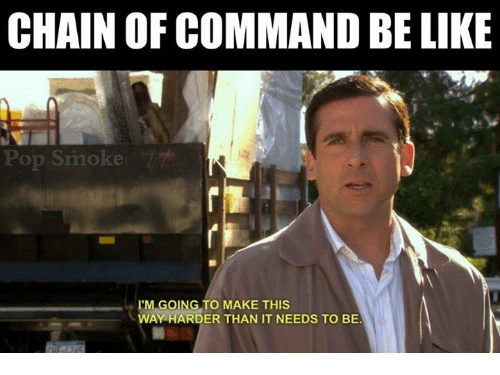
The idea is pretty simple, but the coding goes around the nose.
The flow of performance on the technical side is, you make a physical command, which implements the Control interface, asking the receiver to complete an action, and send the control to the invoker. The invoker is the person that knows when to give this control. The chef is the only i who knows what to practise when given the specific control/social club. So, when the execute method of the invoker is run, it, in turn, causes the command objects' execute method to run on the receiver, thus completing necessary actions.
What we demand to implement is;
- An interface Command
- A course Society that implements Control interface
- A class Waiter (invoker)
- A form Chef (receiver)
And so, the coding goes similar this:
Chef, the receiver
public class Chef { public void cookPasta() { System.out.println("Chef is cooking Craven Alfredo…"); } public void bakeCake() { System.out.println("Chef is baking Chocolate Fudge Cake…"); } }
Command, the interface
public interface Control { public abstract void execute(); }
Guild, the concrete command
public grade Club implements Command { private Chef chef; individual String food; public Order(Chef chef, String food) { this.chef = chef; this.food = food; } @Override public void execute() { if (this.nutrient.equals("Pasta")) { this.chef.cookPasta(); } else { this.chef.bakeCake(); } } }
Waiter, the invoker
public class Waiter { private Order society; public Waiter(Order ord) { this.order = ord; } public void execute() { this.lodge.execute(); } }
Y'all, the client
public class Client { public static void main(String[] args) { Chef chef = new Chef(); Order society = new Club(chef, "Pasta"); Waiter waiter = new Waiter(order); waiter.execute(); order = new Order(chef, "Cake"); waiter = new Waiter(order); waiter.execute(); } }
As you can see to a higher place, the Client makes an Order and sets the Receiver as the Chef. The Gild is sent to the Waiter, who will know when to execute the Society (i.e. when to give the chef the lodge to melt). When the invoker is executed, the Orders' execute method is run on the receiver (i.eastward. the chef is given the control to either cook pasta ? or broil cake?).

Quick recap
In this mail service we went through:
- What a design pattern really is,
- The different types of design patterns and why they are dissimilar
- One basic or mutual pattern design for each type
I promise this was helpful.
Notice the code repo for the postal service, here.
Learn to code for free. freeCodeCamp's open up source curriculum has helped more than forty,000 people get jobs equally developers. Become started
What Level Of Behaviors Deals With Creating Multiple Instances Of A Service To Process Requests?,
Source: https://www.freecodecamp.org/news/the-basic-design-patterns-all-developers-need-to-know/
Posted by: kylelinsomont.blogspot.com
0 Response to "What Level Of Behaviors Deals With Creating Multiple Instances Of A Service To Process Requests?"
Post a Comment